In my last post, I gave a brief overview of what happens when you click the Export Project button in Anthologize, with an eye toward demonstrating the role that third party plugins (written by people Just Like You!) can play in extending the software. In this post, I’ll flesh out some of those details.
Step 1: Anthologize, meet my format
Anthologize has what I like to think of as two internal APIs: the WordPress-facing API, and the theming API. The first step is to let Anthologize know that your format exists, which you’ll do by using the key function in the WP-facing API: anthologize_register_format()
. (Check out how the WPAPI functions work in the source.) You should call anthologize_register_format()
in a function hooked to anthologize_init
, which ensures that it won’t fire before Anthologize is ready for it. Here’s a simple example:
[code language=”php”]
function register_my_anthologize_format() {
$name = ‘example_format’;
$label = ‘My Anthologize Format’;
$loader_file = dirname(__FILE__) . ‘/base.php’;
anthologize_register_format( $name, $label, $loader_file );
}
add_action( ‘anthologize_init’, ‘register_my_anthologize_format’ );
[/code]
anthologize_register_format_option()
takes three arguments. The first is the name that Anthologize will use internally as a unique key for your format. The second is the name that users will see on the Export screen radio button and elsewhere in the interface. (It’s a good idea to set a textdomain for your strings so that they can be translated – eg $label = __( 'My Anthologize Format', 'my-anthologize-plugin' );
.) The third argument is the absolute server path of your main translator file, the file that will be loaded at export time.
You can read a more in-depth example, embedded in a loader class, in this bare-bones plugin that I built for testing purposes. And the definitive examples, of course, can be found in the way that Anthologize registers its native export formats (yum, we love eating our own dog food!).
What does this give you? First, it makes your format available as a format option on the second screen of the export process:
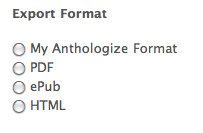
Second, it gives your format a Publishing Options page. Since we haven’t registered any options yet, we get the bare minimum, the shortcode toggle that all formats get:
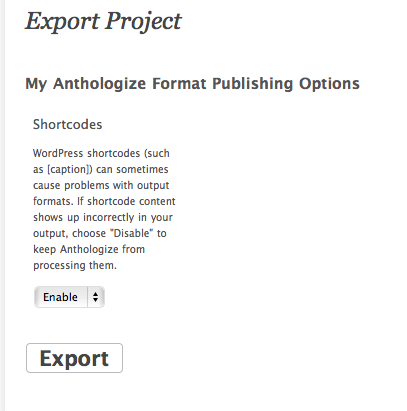
Third – and this is the big thing – anthologize_register_format()
tells Anthologize that, if the user has selected your format on the export screens, the TEI document containing the project data will be handed off to the translator file whose path you specified when you registered the format. That’s where the format translation itself happens.
Step 2: Gimme some options
One of the big thoughts behind the Anthologize plugin architecture is to model WordPress’s design philosophy, which is to provide relatively few options in the core product but to allow for arbitrarily extended functionality by plugins. That’s the purpose behind anthologize_register_format_option()
, the second pillar of Anthologize’s WP-facing API. anthologize_register_format_option()
gives a framework for plugins to register any kind of option associated with their custom format, provides all the integrated markup for presenting those options to users, and passes along the user’s choices to the Anthologize TEI document used for format translation.
Format options can also be loaded at anthologize_init
, though you should be careful to load them after the format itself. I’ll do that by using an add_action
priority:
[code language=”php”]
function register_my_anthologize_format_options() {
$website_name = ‘website’;
$website_label = ‘Website’;
$website_type = ‘textbox’;
anthologize_register_format_option( ‘example_format’, $website_name, $website_label, $website_type );
}
add_action( ‘anthologize_init’, ‘register_my_anthologize_format_options’, 15 );
[/code]
(Note that the first argument is the unique $name of the format I intend to associate the option with.) This registers a plain textbox with the label ‘Website’:
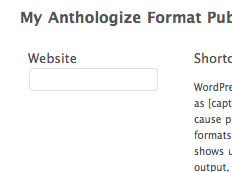
You can also register checkboxes, if you need a Boolean:
[code language=”php”]
$option_name = ‘include_dedication’;
$option_label = ‘Include Dedication?’;
$option_type = ‘checkbox’;
anthologize_register_format_option( ‘example_format’, $option_name, $option_label, $option_type );
[/code]
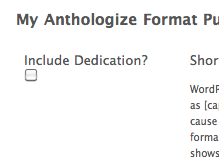
Or you can register dropdowns:
[code language=”php”]
$fontface_format_name = ‘example_format’;
$fontface_option_name = ‘font-face’;
$fontface_label = ‘Font Face’;
$fontface_type = ‘dropdown’;
$fontface_values = array(
‘courier’ => ‘Courier’,
‘georgia’ => ‘Georgia’,
‘garamond’ => ‘Garamond’
);
$fontface_default = ‘georgia’;
anthologize_register_format_option( $fontface_format_name, $fontface_option_name, $fontface_label, $fontface_type, $fontface_values, $fontface_default );
[/code]
This kind of option highlights some of the other possible arguments that anthologize_register_format_option()
will take: an array of possible values, and a default selected value:
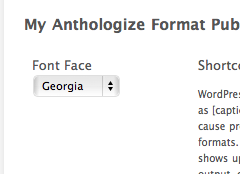
All the options you provide, and in particular the options selected by the user at export time, are handed to your main translator file at the very end of the Anthologize export process.
So that’s how you get your format and its options registered on the WordPress side of things. Stay tuned for a discussion of how translators might be built.
Are you a potential plugin developer with ideas about how this API might evolve to better suit your purposes? Hop onto the dev list.