Google killed Reader just over five years ago. This week, Digg decided to mark this Wood Anniversary by taking a club to its own Reader. Yet again, we oldsters find ourselves shaking our fists at Kids Today. See, for example, Bryan Alexander's thoughtful post on finding a way forward for RSS readers and RSS in general.
I celebrated this turn of events by struggling to set up an instance of Tiny Tiny RSS on my web server. Having finally figured it out, I'm pretty pleased with the functionality. One nice bit is the notice regarding "update errors":
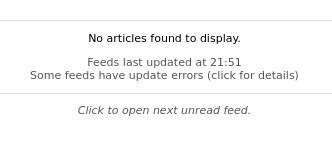
TT-RSS then has a tool for viewing a list of broken feeds. Looking through this list has been an interesting experience. For one thing, it's given me the opportunity to think about a few dozen people who have not otherwise entered my consciousness in a number of years.
More importantly, it's convinced me that the real threat to RSS is not the lack of reader software; you only need a couple of good ones to support an ecosystem. The bigger issue is that RSS content is disappearing.
The more mundane kind of problem is link rot and broken redirects. Many domains have gone away, some have moved in such a way that RSS links no longer work, some are now hidden behind password protection (Blogspot seems to be a particular offender). Web sites come and web sites go, so some of this is to be expected.
The more worrisome trend is content that's not available through RSS simply because there's no feed mechanism. A shamefully large number of my geekier aquantainces have moved their blogs to Jekyll and other static-site-generation tools, which don't appear to have feed support out of the box; and – this is the "shameful" part – since these folks, geeky as they may be, think so little of RSS, they don't bother setting up the secondary plugins or whatever necessary to serve feeds. I expect that kind of behavior from lock-up-my-content companies and technically-clueless organizations that rely heavily on proprietary and bespoke software, but not from people who ought to know better.
For all of its lumbering bloatedness, one of the truly wonderful things about CMSes like WordPress is that they give you things like RSS – along with a pile of other boring-but-critical-to-the-future-of-the-open-web tools – by default. You don't need to make the decision to support RSS readers (or responsive images, or markup that is accessible to assistive technologies, etc) – the system provides them for you, and you have to go out of your way to turn them off.
Those who build their own systems for old-school things like bloggish content distribution, or who rely on teh new hotness to do these tasks in ways that are slicker than the old-school tools, should beware the dangers of discarding the automated systems that are the result of many years and many minds and many mistakes. If you must reinvent the wheel, then do your due diligence. RSS feeds, like other assistive technologies, should not be an afterthought.